|
RSS Feed - WebSphere MQ Support
|
RSS Feed - Message Broker Support
|
 |
|
How Do I Find Channel Status Out in C ? |
« View previous topic :: View next topic » |
Author |
Message
|
debugme |
Posted: Wed Jan 14, 2004 5:43 am Post subject: How Do I Find Channel Status Out in C ? |
|
|
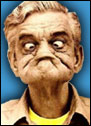 Apprentice
Joined: 14 Jan 2004 Posts: 27
|
Dear Friends,
I want to find out the status of each channel in WebSphere MQ.
Unfortunately it does not seem to be working. Any help would
be much appreciated.
Code: |
mqAddString(requestsBag, MQCACH_CHANNEL_NAME, MQBL_NULL_TERMINATED, "*", &completionCode, &reasonCode);
mqAddInteger(requestsBag, MQIACH_CHANNEL_TYPE, MQIACF_ALL, &completionCode, &reasonCode);
mqExecute(connection, MQCMD_INQUIRE_CHANNEL_STATUS, MQHB_NONE, requestsBag, responseBag, MQHO_NONE, MQHO_NONE,
&completionCode, &reasonCode);
mqCountItems(responseBag, MQHA_BAG_HANDLE, &itemCountInBag, &completionCode, &reasonCode);
for(int count = 0; count < itemCountInBag; ++count)
{
mqInquireBag(responseBag, MQHA_BAG_HANDLE, count,&qAttrsBag, &completionCode, &reasonCode);
mqInquireInteger(qAttrsBag, MQIACH_CHANNEL_STATUS,MQIND_NONE, &channelStatus, &completionCode, &reasonCode);
printf("Status = %d\n", channelStatus);
}
|
|
|
Back to top |
|
 |
jefflowrey |
Posted: Wed Jan 14, 2004 6:11 am Post subject: |
|
|
Grand Poobah
Joined: 16 Oct 2002 Posts: 19981
|
It's not working?
How do you know? _________________ I am *not* the model of the modern major general. |
|
Back to top |
|
 |
clindsey |
Posted: Wed Jan 14, 2004 6:25 am Post subject: |
|
|
Knight
Joined: 12 Jul 2002 Posts: 586 Location: Dallas, Tx
|
Here is some code that I know works. You can build on it to add more attributes to the query as needed. At the time I did it, I was interested only in bytes sent/received.
Be sure you command server is running!
Hope this helps,
Charlie
Code: |
/******************************************************************************/
/* Includes */
/******************************************************************************/
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <ctype.h>
#include <cmqc.h> /* MQI */
#include <cmqcfc.h> /* PCF */
#include <cmqbc.h> /* MQAI */
#define WAIT_TIME 5000 /* wait time for mqexecute */
/******************************************************************************/
/* Function prototypes */
/******************************************************************************/
void CheckCallResult(MQCHAR *, MQLONG , MQLONG);
/******************************************************************************/
/* Function: main */
/******************************************************************************/
int main(int argc, char *argv[])
{
/***************************************************************************/
/* MQAI variables */
/***************************************************************************/
MQHCONN hConn; /* handle to MQ connection */
MQCHAR qmName[MQ_Q_MGR_NAME_LENGTH+1]=""; /* default QMgr name */
MQCHAR cName[MQ_CHANNEL_NAME_LENGTH+1]=""; /* channel name to query */
MQLONG reason; /* reason code */
MQLONG connReason; /* MQCONN reason code */
MQLONG compCode; /* completion code */
MQHBAG adminBag = MQHB_UNUSABLE_HBAG; /* admin bag for mqExecute */
MQHBAG responseBag = MQHB_UNUSABLE_HBAG; /* response bag for mqExecute */
MQHBAG optionsBag = MQHB_UNUSABLE_HBAG; /* options bag for mqExecute */
MQHBAG qAttrsBag; /* bag containing q attributes */
MQHBAG errorBag; /* bag containing cmd server error */
MQLONG mqExecuteCC; /* mqExecute completion code */
MQLONG mqExecuteRC; /* mqExecute reason code */
MQLONG strAttrLength; /* Actual length of q name */
MQLONG intAttr; /* integer attribute returned */
MQLONG i; /* loop counter */
MQLONG numberOfBags; /* number of bags in response bag */
MQCHAR strAttr[256]; /* string attribute returned */
if (argc < 2)
{
printf("Syntax: %s chlname <queue manager name>\n",argv[0]);
printf("\tchlname may contain wildcard characters.\n");
exit(-1);
}
strncpy(cName, argv[1], MQ_CHANNEL_NAME_LENGTH);
printf("\nDisplay current status of channel %s\n\n", cName);
/***************************************************************************/
/* Connect to the queue manager */
/***************************************************************************/
if (argc > 2)
strncpy(qmName, argv[2], (size_t)MQ_Q_MGR_NAME_LENGTH);
MQCONN(qmName, &hConn, &compCode, &connReason);
/***************************************************************************/
/* Report the reason and stop if the connection failed. */
/***************************************************************************/
if (compCode == MQCC_FAILED)
{
CheckCallResult("Queue Manager connection", compCode, connReason);
exit( (int)connReason);
}
/***************************************************************************/
/* Create an admin bag for the mqExecute call */
/***************************************************************************/
mqCreateBag(MQCBO_ADMIN_BAG, &adminBag, &compCode, &reason);
CheckCallResult("Create admin bag", compCode, reason);
/***************************************************************************/
/* Create a response bag for the mqExecute call */
/***************************************************************************/
if (compCode == MQCC_OK)
{
mqCreateBag(MQCBO_ADMIN_BAG, &responseBag, &compCode, &reason);
CheckCallResult("Create response bag", compCode, reason);
}
/***************************************************************************/
/* Create an options bag to set the timeout value of the mqexecute call */
/***************************************************************************/
if (compCode == MQCC_OK)
{
mqCreateBag(MQCBO_USER_BAG, &optionsBag, &compCode, &reason);
CheckCallResult("Create options bag", compCode, reason);
if (compCode == MQCC_OK && reason == MQRC_NONE)
{
mqAddInteger(optionsBag, MQIACF_WAIT_INTERVAL, WAIT_TIME, &compCode, &reason);
}
/********************************************************/
/* If generating the options bag fails, just use the */
/* default time of 30 seconds by setting options bag */
/* to MQHB_NONE */
/********************************************************/
if (compCode != MQCC_OK)
{
optionsBag = MQHB_NONE;
compCode = MQCC_OK;
reason = MQRC_NONE;
}
}
/***************************************************************************/
/* Put the channel name into the admin bag */
/***************************************************************************/
if (compCode == MQCC_OK)
{
mqAddString(adminBag, MQCACH_CHANNEL_NAME, MQBL_NULL_TERMINATED, cName, &compCode, &reason);
CheckCallResult("Add chl name", compCode, reason);
}
/***************************************************************************/
/* Put the instance type into the admin bag */
/* "Current" is the default so this is more for documentation */
/***************************************************************************/
if (compCode == MQCC_OK)
{
mqAddInteger(adminBag, MQIACH_CHANNEL_INSTANCE_TYPE, MQOT_CURRENT_CHANNEL, &compCode, &reason);
CheckCallResult("Add channel instance type", compCode, reason);
}
/***************************************************************************/
/* Indicate we want all attributes */
/***************************************************************************/
if (compCode == MQCC_OK)
{
mqAddInteger(adminBag, MQIACH_CHANNEL_INSTANCE_ATTRS, MQIACF_ALL, &compCode, &reason);
CheckCallResult("Add status attrs", compCode, reason);
}
/***************************************************************************/
/* The mqExecute call creates the PCF structure required, sends it to */
/* the command server, and receives the reply from the command server into */
/* the response bag. The attributes are contained in system bags that are */
/* embedded in the response bag, one set of attributes per bag. */
/***************************************************************************/
if (compCode == MQCC_OK)
{
mqExecute(hConn, /* MQ connection handle */
MQCMD_INQUIRE_CHANNEL_STATUS,/* Command to be executed */
optionsBag, /* No options bag */
adminBag, /* Handle to bag containing commands */
responseBag, /* Handle to bag to receive the response*/
MQHO_NONE, /* Put msg on SYSTEM.ADMIN.COMMAND.QUEUE*/
MQHO_NONE, /* Create a dynamic q for the response */
&compCode, /* Completion code from the mqexecute */
&reason); /* Reason code from mqexecute call */
}
/***************************************************************************/
/* Check the command server is started. If not exit. */
/***************************************************************************/
if (reason == MQRC_CMD_SERVER_NOT_AVAILABLE)
{
printf("Please start the command server: <strmqcsv QMgrName>\n");
MQDISC(&hConn, &compCode, &reason);
CheckCallResult("Disconnect from Queue Manager", compCode, reason);
exit(98);
}
/***************************************************************************/
/* Check the result from mqExecute call. If successful find a display */
/* a few channel attributes. If failed find the error. */
/***************************************************************************/
if ( compCode == MQCC_OK ) /* Successful mqExecute */
{
/*************************************************************************/
/* Count the number of system bags embedded in the response bag from the */
/* mqExecute call. The attributes for each queue are in a separate bag. */
/*************************************************************************/
mqCountItems(responseBag, MQHA_BAG_HANDLE, &numberOfBags, &compCode, &reason);
CheckCallResult("Count number of bag handles", compCode, reason);
for ( i=0; i<numberOfBags; i++)
{
/***********************************************************************/
/* Get the next system bag handle out of the mqExecute response bag. */
/* This bag contains the channel attributes */
/***********************************************************************/
mqInquireBag(responseBag, MQHA_BAG_HANDLE, i, &qAttrsBag, &compCode, &reason);
CheckCallResult("Get the result bag handle", compCode, reason);
if (compCode == MQCC_OK && reason == MQRC_NONE)
{
/***********************************************************************/
/* Get the channel name out of the channel attributes bag */
/***********************************************************************/
mqInquireString(qAttrsBag, MQCACH_CHANNEL_NAME, 0, MQ_CHANNEL_NAME_LENGTH, strAttr,
&strAttrLength, NULL, &compCode, &reason);
if (compCode == MQCC_OK && reason == MQRC_NONE)
{
mqTrim(MQ_CHANNEL_NAME_LENGTH, strAttr, strAttr, &compCode, &reason);
printf("Channel name: %s\n", strAttr);
}
/***********************************************************************/
/* Get the channel status out of the attributes bag */
/***********************************************************************/
mqInquireInteger(qAttrsBag, MQIACH_CHANNEL_STATUS, MQIND_NONE, &intAttr,
&compCode, &reason);
if (compCode == MQCC_OK && reason == MQRC_NONE)
{
printf("Channel Status: ");
switch (intAttr)
{
case MQCHS_BINDING:
printf("Binding\n");
break;
case MQCHS_STARTING:
printf("Starting\n");
break;
case MQCHS_RUNNING:
printf("Running\n");
break;
case MQCHS_PAUSED:
printf("Paused\n");
break;
case MQCHS_STOPPING:
printf("Stopping\n");
break;
case MQCHS_RETRYING:
printf("Retrying\n");
break;
case MQCHS_STOPPED:
printf("Stopped\n");
break;
case MQCHS_REQUESTING:
printf("Requesting\n");
break;
case MQCHS_INITIALIZING:
printf("Initializing\n");
break;
default:
printf("UNKNOWN\n");
}
}
/***********************************************************************/
/* Get the Number of Messages Sent out of the attributes bag */
/***********************************************************************/
mqInquireInteger(qAttrsBag, MQIACH_MSGS, MQIND_NONE, &intAttr,
&compCode, &reason);
if (compCode == MQCC_OK && reason == MQRC_NONE)
{
printf("Number of Messages Sent: %d\n",intAttr);
}
/***********************************************************************/
/* Get the Number of Bytes Sent out of the attributes bag */
/***********************************************************************/
mqInquireInteger(qAttrsBag, MQIACH_BYTES_SENT, MQIND_NONE, &intAttr,
&compCode, &reason);
if (compCode == MQCC_OK && reason == MQRC_NONE)
{
printf("Number of Bytes Sent: %u\n",(unsigned long)intAttr);
}
/***********************************************************************/
/* Get the Number of Bytes Received out of the attributes bag */
/***********************************************************************/
mqInquireInteger(qAttrsBag, MQIACH_BYTES_RCVD, MQIND_NONE, &intAttr,
&compCode, &reason);
if (compCode == MQCC_OK && reason == MQRC_NONE)
{
printf("Number of Bytes Received: %d\n",intAttr);
}
}
printf("\n");
}
}
else /* Failed mqExecute */
{
/*************************************************************************/
/* If the command fails get the system bag handle out of the mqexecute */
/* response bag.This bag contains the reason from the command server */
/* why the command failed. */
/*************************************************************************/
switch (reason)
{
case MQRCCF_COMMAND_FAILED:
mqInquireBag(responseBag, MQHA_BAG_HANDLE, 0, &errorBag, &compCode, &reason);
CheckCallResult("Get the result bag handle", compCode, reason);
/***********************************************************************/
/* Get the completion code and reason code, returned by the command */
/* server, from the embedded error bag. */
/***********************************************************************/
mqInquireInteger(errorBag, MQIASY_COMP_CODE, MQIND_NONE, &mqExecuteCC,
&compCode, &reason );
CheckCallResult("Get the completion code from the result bag", compCode, reason);
mqInquireInteger(errorBag, MQIASY_REASON, MQIND_NONE, &mqExecuteRC,
&compCode, &reason);
CheckCallResult("Get the reason code from the result bag", compCode, reason);
if (mqExecuteRC == MQRCCF_CHL_STATUS_NOT_FOUND)
{
printf("No status available for channel %s.\n",cName);
}
else
{
printf("Error returned by the command server: Completion Code = %d : Reason = %d\n",
mqExecuteCC, mqExecuteRC);
}
break;
case MQRC_NO_MSG_AVAILABLE:
printf("No results returned for channel %s. This channel may not exist.\n", cName);
break;
default:
printf("Call to get channel status attributes failed: Completion Code = %d : Reason = %d\n",
compCode, reason);
}
}
/***************************************************************************/
/* Delete the admin bag if successfully created. */
/***************************************************************************/
if (adminBag != MQHB_UNUSABLE_HBAG)
{
mqDeleteBag(&adminBag, &compCode, &reason);
CheckCallResult("Delete the admin bag", compCode, reason);
}
/***************************************************************************/
/* Delete the response bag if successfully created. */
/***************************************************************************/
if (responseBag != MQHB_UNUSABLE_HBAG)
{
mqDeleteBag(&responseBag, &compCode, &reason);
CheckCallResult("Delete the response bag", compCode, reason);
}
/***************************************************************************/
/* Disconnect from the queue manager if not already connected */
/***************************************************************************/
if (connReason != MQRC_ALREADY_CONNECTED)
{
MQDISC(&hConn, &compCode, &reason);
CheckCallResult("Disconnect from Queue Manager", compCode, reason);
}
return MQCC_OK;
}
/******************************************************************************/
/* */
/* Function: CheckCallResult */
/* */
/******************************************************************************/
/* */
/* Input Parameters: Description of call */
/* Completion code */
/* Reason code */
/* */
/* Output Parameters: None */
/* */
/* Logic: Display the description of the call, the completion code and the */
/* reason code if the completion code is not successful */
/* */
/******************************************************************************/
void CheckCallResult(char *callText, MQLONG cc, MQLONG rc)
{
if (cc != MQCC_OK)
printf("%s failed: Completion Code = %d : Reason = %d\n", callText, cc, rc);
}
|
|
|
Back to top |
|
 |
debugme |
Posted: Wed Jan 14, 2004 7:10 am Post subject: |
|
|
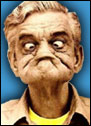 Apprentice
Joined: 14 Jan 2004 Posts: 27
|
Dear Charlie,
Thanks for the source code. I modified it to make use of my own queue manager and channel name. But when I ran it, I got the message
Code: |
No status available for channel |
However, another program I wrote earlier, tells me that I have the following channels on my system
Code: |
Channel Name = CHAN1
Channel Name = SYSTEM.ADMIN.SVRCONN
Channel Name = SYSTEM.AUTO.RECEIVER
Channel Name = SYSTEM.AUTO.SVRCONN
Channel Name = SYSTEM.DEF.CLUSRCVR
Channel Name = SYSTEM.DEF.CLUSSDR
Channel Name = SYSTEM.DEF.RECEIVER
Channel Name = SYSTEM.DEF.REQUESTER
Channel Name = SYSTEM.DEF.SENDER
Channel Name = SYSTEM.DEF.SERVER
Channel Name = SYSTEM.DEF.SVRCONN
Channel Name = S_itrspc48
Channel Name = TO_QM_itrspc48
Channel Name = CHAN2
Channel Name = SYSTEM.DEF.CLNTCONN
|
Any idea what is going on ? I'm pretty stumped, as no doubt my avatar indicates !
regards, Asad. |
|
Back to top |
|
 |
JasonE |
Posted: Wed Jan 14, 2004 8:18 am Post subject: |
|
|
Grand Master
Joined: 03 Nov 2003 Posts: 1220 Location: Hursley
|
You only have channel status entries for running, retrying, stopped etc channels. Once the channel goes back to its default state (inactive), there is no channel status entry.
Use runqmsc "dis chs(*) all" to see what I mean. |
|
Back to top |
|
 |
debugme |
Posted: Wed Jan 14, 2004 8:45 am Post subject: |
|
|
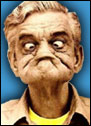 Apprentice
Joined: 14 Jan 2004 Posts: 27
|
Jason,
Thanks very much for the clarification
But is there any way in which I can get a list of all the inactive channels ?
I would appreciate any code snippets in C.
regards, Asad. |
|
Back to top |
|
 |
JasonE |
Posted: Wed Jan 14, 2004 9:06 am Post subject: |
|
|
Grand Master
Joined: 03 Nov 2003 Posts: 1220 Location: Hursley
|
Not easily (that I know of!).
Get a list of all channels and compare this to a list of all channel status' (or is it stati?) might work. The GUI (Explorer) certainly works similar to that as I understand it. |
|
Back to top |
|
 |
debugme |
Posted: Wed Jan 14, 2004 2:46 pm Post subject: |
|
|
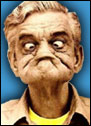 Apprentice
Joined: 14 Jan 2004 Posts: 27
|
Let me get this straight...You're suggesting I get a list of channel names and then try to get the status of each. For those channels where I can get the status, the implication is that they are active. For those where I cannot get the status, the implication is that they are inactive.
That actually makes sense.
Thanks for getting back to me on that
regards, Asad. |
|
Back to top |
|
 |
JasonE |
Posted: Thu Jan 15, 2004 2:22 am Post subject: |
|
|
Grand Master
Joined: 03 Nov 2003 Posts: 1220 Location: Hursley
|
Hmm let me be slightly more precise - There is no 'good' way of doing this (that I know of) when you get to a system with thousands of channels. For smaller systems, that will work fine.
If you did PCF equiv to DIS CHL(*) and DIS CHS(*), and joined them in your code, you have 2 outbound messages and a number of inbound ones.
If you did PCF equiv to DIS CHL(*) and an individual DIS CHS(chlname) per channel, you have lots of outbound messages and lots of inbound ones.
Therefore as the system gets larger, the number of messages flowing gets large, and the impact of locking the status table repeatedly can impact the system if you thrash the server with these DIS CHS(*) commands. |
|
Back to top |
|
 |
|
|
 |
|
Page 1 of 1 |
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|
|
|